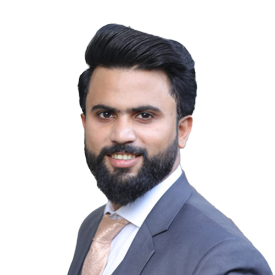
Software Engineer Dynamics 365 F&O
Subscribe to the newsletter
Number sequence is a feature in Microsoft Dynamics 365 Finance and Operations that enables the system to generate a unique identifier for records automatically. It lets you to define a specific format for the identifier, tailored to the type of record being created. This feature is particularly useful in situations where a unique value is needed but no predefined identifier is available.
Number sequences in Dynamics 365 Finance and Operations play a crucial role in organizing and categorizing transactions. They help maintain accurate records, streamline data retrieval, and improve overall system efficiency.
In this blog, we’ll explore what number sequences are, how they enhance transaction management, and provide a step-by-step guide to setting them up in Dynamics 365 Finance and Operations.
What are number sequences?
Number sequences in Dynamics 365 Finance and Operations are unique identifiers assigned to records such as invoices, purchase orders, and customers. They help organize and categorize transactions, ensuring consistency and easy retrieval of data. By defining number sequences, businesses can streamline record-keeping and maintain structured, traceable information across the system.
Example of a number sequence
For example, when dealing with student IDs, educational institutions may have a predefined numbering system for identifying students. However, in many other cases, such as when creating new student records or enrollments, it’s more efficient to have the system generate a unique identifier. Using number sequences, Dynamics 365 Finance and Operations saves time and ensures consistency as the system automatically provides a unique student ID whenever one is needed, eliminating the need for manual input.
Disclaimer: This is just an illustrative example for better understanding. Microsoft Dynamics 365 does not provide any specific module or form for educational institutions, and this example does not imply that such feature exists in the platform.
Getting started with number sequence in Dynamics 365 Finance and Operations
To develop a number sequence in Microsoft Dynamics 365 F&O, several key steps must be followed. Below is a high-level overview of the necessary steps, followed by a more detailed explanation of each one.
- First, identify the field, table, and form that will use the number sequence.
- Next, create a class that extends the NumberSeqApplicationModule. This class will include the code required to add a row to the ‘Number sequence’ tab on the parameters form. The ‘number sequence reference’ allows the user to specify which ‘number sequence code’ should be used for the selected field.
- Then, execute a runnable class (job) to process the class created in the previous step. This action will generate the ‘number sequence reference’ row.
- After this, assign a ‘number sequence code’ to the ‘number sequence reference,’ defining the format of the number sequence.
- Finally, override the ‘create’ method in the data source of the form that will utilize the number sequence. This method should include code to fetch a new number from the assigned number sequence each time a new record is created.
Now let’s look into each step in detail.
Step 1: Identify the field
First, we need to determine which field on a table should be assigned to use a number sequence.
Suppose the table contains two fields: StudentId and Description. The StudentId field has the Extended Data Type (EDT) property set to ‘StudentId.’
Extended Data Types (EDTs) are separate objects created in Visual Studio that define various properties, such as string size and label. Any field on a table that uses a specific EDT will automatically inherit these properties. In this case, the StudentId field inherits the properties defined in the ‘StudentId’ EDT.
Step 2: Create the NumberSeqApplicationModule class
To develop a number sequence in Microsoft Dynamics 365 F&O, start by creating a new class in Visual Studio that extends the NumberSeqApplicationModule. This class will include the necessary code to add a row to the ‘Number sequence’ tab on a parameters form. The ‘number sequence reference’ enables the user to choose the appropriate ‘number sequence code’ for that field.
For instance, I named my number sequence reference ‘ CFMQ_StudentIdNumberSeq‘. The code will look something like this:
class CFMQ_StudentIdNumberSeq extends NumberSeqApplicationModule
{
}
Step 3: Leverage the BuildModulesMapSubscriber method
Next, include the following method and code. While it’s not essential to fully understand the details of this method, its purpose is to add your EDT to the list of number sequence references. Be sure to replace the text ‘classnum(CFMQ_StudentIdNumberSeq)’ with the name of your own class.
[SubscribesTo(classstr(NumberSeqGlobal),delegatestr(NumberSeqGlobal,buildModulesMapDelegate))]
static void buildModulesMapSubsciber(Map numberSeqModuleNamesMap)
{
NumberSeqGlobal::addModuleToMap(classnum(CFMQ_StudentIdNumberSeq), numberSeqModuleNamesMap);
}
Step 4: NumberSeqModule method
Number sequence codes are set up and linked to number sequence references on the parameters forms in each module. Before proceeding, it’s important to determine which ‘Module’ of the system should have this number sequence configured. Typically, the module associated with the menu item that opens the form using the field should be chosen.
In our example, the student form displays data from the student table, and this form is part of the Accounts Receivable module in Microsoft Dynamics 365 Finance and Operations. Therefore, we will add a code that uses the ‘Cust’ value from the ‘NumberSeqModule’ enum.
public NumberSeqModule numberSeqModule()
{
return NumberSeqModule::Cust;
}
It can be a bit tricky to determine which enum corresponds to which module. However, you can check the ‘label’ of the enum in Visual Studio to help you identify the correct match.
Step 5: Define the scope and parameters using the LoadModule method
Lastly, a key step to develop a Microsoft Dynamics 365 for Finance and Operations number sequence is to define what scope and parameters should be used. Add the ‘loadModule‘ method by following the example below. Then, replace ‘StudentId‘ in the example, with the name of your EDT.
Also, add ‘Reference help‘ text that will help the user in knowing which number sequence they are setting up. This should be changed to use a Label, instead of plain text.
protected void loadModule()
{
NumberSeqDatatype datatype = NumberSeqDatatype::construct();
datatype.parmDatatypeId(extendedtypenum(StudentId));
datatype.parmReferenceHelp(literalstr(“Student id”));
datatype.parmWizardIsContinuous(true);
datatype.parmWizardIsManual(NoYes::No);
datatype.parmWizardIsChangeDownAllowed(NoYes::No);
datatype.parmWizardIsChangeUpAllowed(NoYes::No);
datatype.parmWizardHighest(999999);
datatype.parmSortField(1);
datatype.addParameterType(NumberSeqParameterType::DataArea, true, false);
this.create(datatype);
}
To explain, the other parameter values can also be changed based on your needs. However, the above code shows the most common values.
Step 6: Add the final NumberSeqApplicationModule class
In the end, your class for developing a Microsoft Dynamics 365 Finance and Operations number sequence should resemble the structure below. We have highlighted the sections that need to be customized for your specific scenario.
class CFMQ_StudentIdNumberSeq extends NumberSeqApplicationModule
{
#Define.MAXSEQRANGE(99999999)
protected void loadModule()
{
NumberSeqDatatype datatype = NumberSeqDatatype::construct();
datatype.parmDatatypeId(extendedtypenum(StudentId));
datatype.parmReferenceHelp(literalstr("Student id"));
datatype.parmWizardIsContinuous(true);
datatype.parmWizardIsManual(NoYes::No);
datatype.parmWizardIsChangeDownAllowed(NoYes::No);
datatype.parmWizardIsChangeUpAllowed(NoYes::No);
datatype.parmWizardHighest(999999);
datatype.parmSortField(1);
datatype.addParameterType(NumberSeqParameterType::DataArea, true, false);
this.create(datatype);
}
public NumberSeqModule numberSeqModule()
{
return NumberSeqModule::Cust;
}
[SubscribesTo(classstr(NumberSeqGlobal),delegatestr(NumberSeqGlobal,buildModulesMapDelegate))]
static void buildModulesMapSubsciber(Map numberSeqModuleNamesMap)
{
NumberSeqGlobal::addModuleToMap(classnum(CFMQ_StudentIdNumberSeq), numberSeqModuleNamesMap);
}
}
Step 7: Create runnable class (Job)
After creating the new class that extends NumberSeqApplicationModule, the next step is to run the LoadModule method within it. This will generate a record in the ‘Number sequence’ tab of the parameters form in the specified module.
Here’s how you can do it:
- Open Visual Studio and create a new solution and project.
- Right-click on the project, and select Add > New Item.
- In the Add New Item window, choose Dynamics 365 Items on the left, and then select Runnable Class (Job) from the list.
- Enter a name for the runnable class (job).
- Click Add to add the job to your project.
For example, we named our job LoadStudentIdNumSeqJob:
internal final class LoadStudentIdNumSeqJob
{
/// /// Class entry point. The system will call this method when a designated menu /// is selected or when execution starts and this class is set as the startup class. ///
/// The specified arguments.
public static void main(Args _args)
{
CFMQ_StudentIdNumberSeq seq = new CFMQ_StudentIdNumberSeq();
seq.load();
}
Notice that in this step, we are simply instantiating the class created in the previous step and then calling the Load method. This method is available in the base class, and it will trigger the LoadModule method in the child class.
Once that’s done, right-click the class in your Visual Studio project and select Set as Startup Object. This ensures that the runnable class is executed when you run the project.
Finally, click the Start button in Visual Studio to run the job. This will execute the LoadModule method, creating the number sequence reference as intended.
Step 8: Set the D365 number sequence reference
After running the job, navigate to the parameters form where you added the number sequence reference. Then, go to the number sequence tab and verify that a new row has been created, showing the number sequence reference you set up.
In our example, we can see the new number sequence reference appear on the Accounts receivable parameters form.
Next, if you already have a number sequence created, you can populate the number sequence reference with a number sequence code. If not, navigate to Organization administration > Number sequences > Number sequences, and then click the Generate button to run the wizard and create a new number sequence.
- In the Setup section, you will notice that the system has automatically identified that a number sequence code needs to be created for each company in the system. Click the Next button to proceed.
- Finally, review the information on the Completed page, and click Finish to complete the process.
- Once back on the number sequence list page, use the filters to locate the number sequence code that was generated by the wizard.
Step 9: Override the create method
Up until now, the number sequence was not fully defined and set up for use. Now, we can complete the final step required to develop a Microsoft Dynamics 365 Finance and Operations number sequence. Whenever a new record is created on our form, we want the system to automatically generate a new unique number.
Here’s how to do it:
- Open the form you are working with, for example, Student.
- Next, right-click on the data source that contains the field tied to the Extended Data Type (EDT) linked to the number sequence.
- Select Override, then choose Create from the context menu.
This will allow you to customize the record creation process to include number sequence generation.
Add the following code to the ‘create‘ method:
public void create(boolean _append = false)
{
element.numberSeqFormHandler().formMethodDataSourceCreatePre();
super(_append);
element.numberSeqFormHandler().formMethodDataSourceCreate();
}
Step 10: Test the number sequence
Now comes the exciting part: testing to ensure the number sequence we set up works as expected. Here is what you have to do:
- After compiling the code, open the form associated with the table that uses the number sequence.
- Click the New button to create a new record.
- The field that uses the number sequence should automatically be populated with a value that follows the format defined by the number sequence, confirming that everything is working correctly.
Summing up
By following the step-by-step process outlined in this guide, you can create and configure number sequences tailored to your business needs. Proper implementation enhances data accuracy, improves transaction tracking, and streamlines operations.
If you need expert guidance on implementing number sequences or optimizing your D365 system, reach out to our team at marketing@confiz.com for tailored solutions.